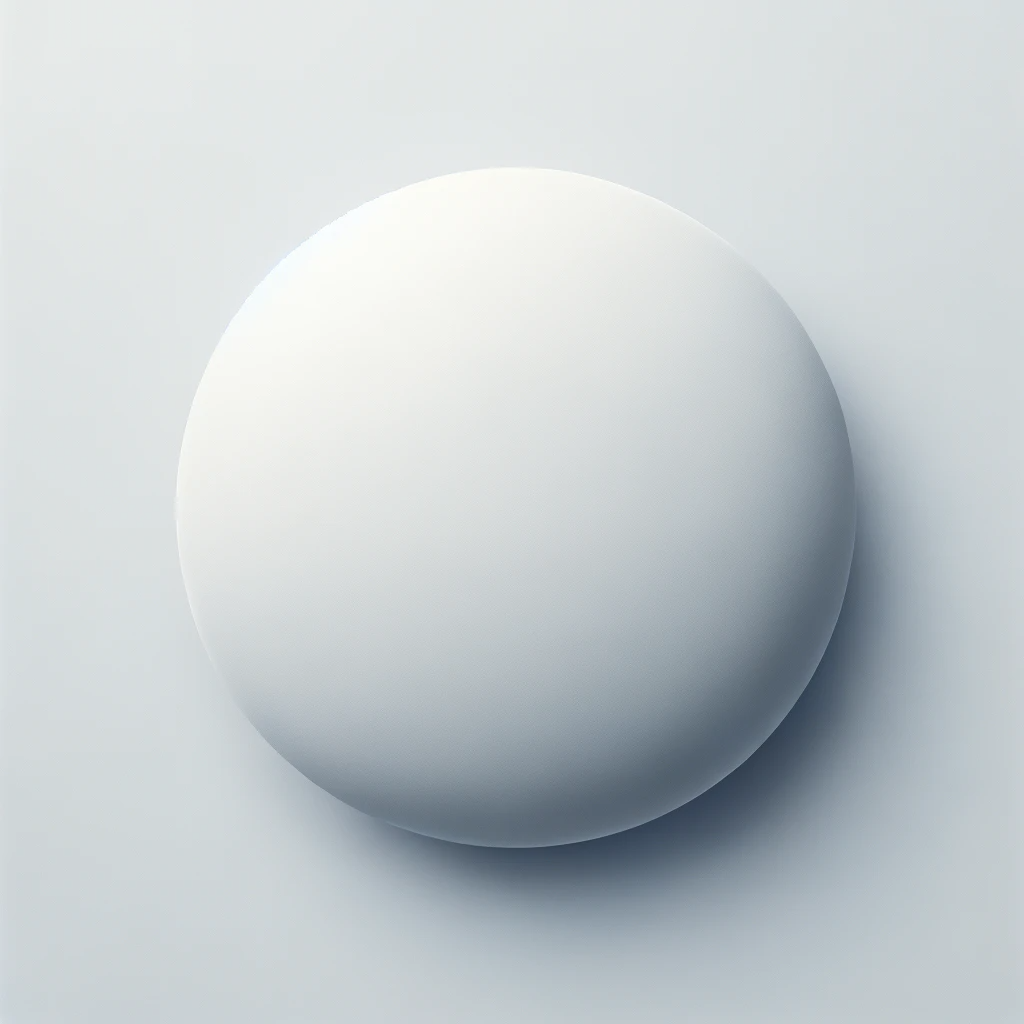
scipy.optimize.newton# scipy.optimize. newton (func, x0, fprime = None, args = (), tol = 1.48e-08, maxiter = 50, fprime2 = None, x1 = None, rtol = 0.0, full_output = False, disp = True) [source] # Find a root of a real or complex function using the Newton-Raphson (or secant or Halley’s) method. Find a root of the scalar-valued function func given a nearby …for standard (LP,QP) and gradient based optimization problems (LBFGS, Proximal Splitting, Projected gradient). As of now it provides the following solvers: Linear Program (LP) solver using scipy, cvxopt, or GUROBI solver.Optimizing Python code is essential for developers looking to create high-performance software, reduce resource consumption, and improve user experience. This article aims to provide intermediate to advanced Python programmers with the latest tips and techniques to help you write faster, more efficient code. By implementing these …3. I have been trying to optimize a python script I wrote for the last two days. Using several profiling tools (cProfile, line_profiler etc.) I narrowed down the issue to the following function below. df is a numpy array with 3 columns and +1,000,000 rows (data type is float). Using line_profiler, I found out that the function spends most of ... Optimization happens everywhere. Machine learning is one example of such and gradient descent is probably the most famous algorithm for performing optimization. Optimization means to find the best value of some function or model. That can be the maximum or the minimum according to some metric. Using clear explanations, standard Python libraries ... May 25, 2022 · Newton’s method for optimization is a particular case of a descent method. With “ f′′ (xk ) ” being the derivative of the derivative of “ f” evaluated at iteration “ k”. Consider ... Jul 16, 2020 · Wikipedia defines optimization as a problem where you maximize or minimize a real function by systematically choosing input values from an allowed set and computing the value of the function. That means when we talk about optimization we are always interested in finding the best solution. Are you an intermediate programmer looking to enhance your skills in Python? Look no further. In today’s fast-paced world, staying ahead of the curve is crucial, and one way to do ...Latest releases: Complete Numpy Manual. [HTML+zip] Numpy Reference Guide. [PDF] Numpy User Guide. [PDF] F2Py Guide. SciPy Documentation.Generally speaking for loop optimization, the more complex loop should be the inner loop (looks correct), and you can vectorize operations. Beyond that you can use some JIT compilers like Numba, and ultimately Cython could improve performance 10 …Learn how to use scipy.optimize package for unconstrained and constrained minimization, least-squares, root finding, and linear programming. See examples of different optimization methods and options for multivariate scalar …Aug 30, 2023 · 4. Hyperopt. Hyperopt is one of the most popular hyperparameter tuning packages available. Hyperopt allows the user to describe a search space in which the user expects the best results allowing the algorithms in hyperopt to search more efficiently. Currently, three algorithms are implemented in hyperopt. Random Search. This paper presents a Python wrapper and extended functionality of the parallel topology optimization framework introduced by Aage et al. (Topology optimization using PETSc: an easy-to-use, fully parallel, open source topology optimization framework. Struct Multidiscip Optim 51(3):565–572, 2015). The Python interface, which simplifies …Apr 6, 2022 ... Since, the initial grid is normalized, meaning each cell is 1 by 1 units in size, you need to multiply the row and column values by the real ...Optimization is the problem of finding a set of inputs to an objective function that results in a maximum or minimum function evaluation. It is the challenging problem that underlies many machine learning algorithms, from fitting logistic regression models to training artificial neural networks. There are perhaps hundreds of popular optimization … Optimization happens everywhere. Machine learning is one example of such and gradient descent is probably the most famous algorithm for performing optimization. Optimization means to find the best value of some function or model. That can be the maximum or the minimum according to some metric. Using clear explanations, standard Python libraries ... Modern society is built on the use of computers, and programming languages are what make any computer tick. One such language is Python. It’s a high-level, open-source and general-...In this article, I will demonstrate solutions to some optimization problems, leveraging on linear programming, and using PuLP library in Python. Linear programming deals with the problem of optimizing a linear objective function (such as maximum profit or minimum cost) subject to linear equality/inequality …This package provides an easy-to-go implementation of meta-heuristic optimizations. From agents to search space, from internal functions to external communication, we will foster all research related to optimizing stuff. Use Opytimizer if you need a library or wish to: Create your optimization algorithm; Design or use pre-loaded optimization tasks;You were correct that my likelihood function was wrong, not the code. Using a formula I found on wikipedia I adjusted the code to: m = parameters[0] b = parameters[1] sigma = parameters[2] for i in np.arange(0, len(x)): y_exp = m * x + b. L = (len(x)/2 * np.log(2 * np.pi) + len(x)/2 * np.log(sigma ** 2) + 1 /. (2 * sigma ** 2) * sum((y - y_exp ...Through these three articles, we learned step by step how to formalize an optimization problem and how to solve it using Python and Gurobi solver. This methodology has been applied to a Make To Order factory that needs to schedule its production to reduce the costs, including labour, inventory, and shortages.The scipy.optimize package provides several commonly used optimization algorithms. A detailed listing is available: scipy.optimize (can also …Pyomo provides a means to build models for optimization using the concepts of decision variables, constraints, and objectives from mathematical optimization, …Linear programming is a powerful tool for helping organisations make informed decisions quickly. It is a useful skill for Data Scientists, and with open-source libraries such as Pyomo it is easy to formulate models in Python. In this post, we created a simple optimisation model for efficiently scheduling surgery cases.The choice of optimization algorithm for your deep learning model can mean the difference between good results in minutes, hours, and days. The Adam optimization algorithm is an extension to stochastic gradient descent that has recently seen broader adoption for deep learning applications in computer vision and natural language processing.. In this post, …Visualization for Function Optimization in Python. By Jason Brownlee on October 12, 2021 in Optimization 5. Function optimization involves finding the input that results in the optimal value from an objective function. Optimization algorithms navigate the search space of input variables in order to locate the optima, and both the shape of the ...Python is a versatile programming language that is widely used for game development. One of the most popular games created using Python is the classic Snake Game. To achieve optima...Running A Portfolio Optimization. The two key inputs to a portfolio optimization are: Expected returns for each asset being considered.; The covariance matrix of asset returns.Embedded in this are information on cross-asset correlations and each asset’s volatility (the diagonals).; Expected returns are hard to estimate — some people … Mathematical optimization: finding minima of functions — Scipy lecture notes. 2.7. Mathematical optimization: finding minima of functions ¶. Mathematical optimization deals with the problem of finding numerically minimums (or maximums or zeros) of a function. In this context, the function is called cost function, or objective function, or ... Portfolio optimization in finance is the technique of creating a portfolio of assets, for which your investment has the maximum return and minimum risk. Investor’s Portfolio Optimization using Python with Practical Examples. Photo by Markus. In this tutorial you will learn: What is portfolio optimization? What does a …Mar 18, 2024 ... In this module, we introduce the concept of optimization, show how to solve mathematical optimization problems in Python and SciPy, ... Our framework offers state of the art single- and multi-objective optimization algorithms and many more features related to multi-objective optimization such as visualization and decision making. pymoo is available on PyPi and can be installed by: pip install -U pymoo. Please note that some modules can be compiled to speed up computations ... Jul 23, 2021 · The notebook illustrates one way of doing this, called a points race. Using HumpDay points_race to assess optimizer performance on a list of objective functions. Maybe that takes too long for your ... optimization, collection of mathematical principles and methods used for solving quantitative problems in many disciplines, including physics, biology, engineering, economics, and business. The subject grew from a realization that quantitative problems in manifestly different disciplines have important mathematical elements in common. Optimizing Python code is essential for developers looking to create high-performance software, reduce resource consumption, and improve user experience. This article aims to provide intermediate to advanced Python programmers with the latest tips and techniques to help you write faster, more efficient code. By implementing these …Performance options ¶. Configuring Python using --enable-optimizations --with-lto (PGO + LTO) is recommended for best performance. The experimental --enable-bolt flag can also be used to improve performance. Enable Profile Guided Optimization (PGO) using PROFILE_TASK (disabled by default).SciPy optimize provides functions for minimizing (or maximizing) objective functions, possibly subject to constraints. It includes solvers for nonlinear … optimization, collection of mathematical principles and methods used for solving quantitative problems in many disciplines, including physics, biology, engineering, economics, and business. The subject grew from a realization that quantitative problems in manifestly different disciplines have important mathematical elements in common. Our framework offers state of the art single- and multi-objective optimization algorithms and many more features related to multi-objective optimization such as visualization and decision making. pymoo is available on PyPi and can be installed by: pip install -U pymoo. Please note that some modules can be compiled to speed up computations ... Here I have compiled 7 useful Python libraries that will help you with Optimization. 1. Hyperopt. This library will help you to optimize the hyperparameters of machine learning models. It is useful for serial and parallel optimization over awkward search spaces, which may include real-valued, discrete, and conditional dimensions.Nov 12, 2023 ... Join the Byte Club to practice your Python skills! ($2.99/mo): https://www.youtube.com/channel/UCTrAO0TDCldnYUN3BkLmGcw/join Follow me on ...RSOME (Robust Stochastic Optimization Made Easy) is an open-source Python package for generic modeling of optimization problems (subject to uncertainty). Models in RSOME are constructed by variables, constraints, and expressions that are formatted as N-dimensional arrays. These arrays are consistent with the NumPy library …scipy.optimize.brute# scipy.optimize. brute (func, ranges, args=(), Ns=20, full_output=0, finish=<function fmin>, disp=False, workers=1) [source] # Minimize a function over a given range by brute force. Uses the “brute force” method, i.e., computes the function’s value at each point of a multidimensional grid of points, to find the global minimum of the function.Oct 5, 2021 ... The mCVAR is another popular alternative to mean variance optimization. It works by measuring the worst-case scenarios for each asset in the ...I am looking to solve the following constrained optimization problem using scipy.optimize Here is the function I am looking to minimize: here A is an m X n matrix , the first term in the minimization is the residual sum of squares, the second is the matrix frobenius (L2 norm) of a sparse n X n matrix W, and the third one is an L1 norm of the ...SciPy optimize provides functions for minimizing (or maximizing) objective functions, possibly subject to constraints. It includes solvers for nonlinear …Mar 11, 2024 · Learn how to use OR-Tools for Python to solve optimization problems in Python, such as linear, quadratic, and mixed-integer problems. Follow the steps to set up and run a simple example of a linear optimization problem with the GLOP solver. scipy.optimize.brute# scipy.optimize. brute (func, ranges, args=(), Ns=20, full_output=0, finish=<function fmin>, disp=False, workers=1) [source] # Minimize a function over a given range by brute force. Uses the “brute force” method, i.e., computes the function’s value at each point of a multidimensional grid of points, to find the global minimum of the function.Feb 22, 2021 ... In this video, I'll show you the bare minimum code you need to solve optimization problems using the scipy.optimize.minimize method.scipy.optimize.OptimizeResult# class scipy.optimize. OptimizeResult [source] #. Represents the optimization result. Notes. Depending on the specific solver being used, OptimizeResult may not have all attributes listed here, and they may have additional attributes not listed here. Since this class is essentially a subclass of …Feb 3, 2023 ... The selection of solver parameters or initial guesses can be determined by another optimization algorithm to search in among categorical or ...According to the Smithsonian National Zoological Park, the Burmese python is the sixth largest snake in the world, and it can weigh as much as 100 pounds. The python can grow as mu...In this article, some interesting optimization tips for Faster Python Code are discussed. These techniques help to produce result faster in a python code. Use builtin functions and libraries: Builtin functions like map () are implemented in C code. So the interpreter doesn’t have to execute the loop, this gives a …GEKKO Python is designed for large-scale optimization and accesses solvers of constrained, unconstrained, continuous, and discrete problems. Problems in linear programming, quadratic programming, integer programming, nonlinear optimization, systems of dynamic nonlinear equations, and multi-objective optimization can be solved. for standard (LP,QP) and gradient based optimization problems (LBFGS, Proximal Splitting, Projected gradient). As of now it provides the following solvers: Linear Program (LP) solver using scipy, cvxopt, or GUROBI solver. Python Code Optimization Tips and Tricks for Geeks. Let’s first begin with some of the core internals of Python that you can exploit to your advantage. 1. Interning Strings for Efficiency. Interning a string is a method of storing only a single copy of each distinct string. And, we can make the Python interpreter reuse strings by manipulating ...The Nelder-Mead optimization algorithm can be used in Python via the minimize () function. This function requires that the “ method ” argument be set to “ nelder-mead ” to use the Nelder-Mead algorithm. It takes the objective function to be minimized and an initial point for the search. 1. 2.Python Software for Convex Optimization . CVXOPT is a free software package for convex optimization based on the Python programming language. It can be used with the interactive Python interpreter, on the command line by executing Python scripts, or integrated in other software via Python extension modules. Its main purpose is to make …It is necessary to import python-scip in your code. This is achieved by including the line. from pyscipopt import Model. Create a solver instance. model = Model("Example") # model name is optional. Access the methods in the scip.pxi file using the solver/model instance model, e.g.: x = model.addVar("x")Optlang is a Python package for solving mathematical optimization problems, i.e. maximizing or minimizing an objective function over a set of variables subject to a number of constraints. Optlang provides a common interface to a series of optimization tools, so different solver backends can be changed in a …Optimization modelling, most of the time used as simply ‘optimization’, is a part of broader research field called Operations Research. In this article I will give brief comparison of three ...Bayesian Optimization provides a probabilistically principled method for global optimization. How to implement Bayesian Optimization from scratch and how to use open-source implementations. Kick-start your project with my new book Probability for Machine Learning, including step-by-step tutorials and the Python source code files for …Feb 3, 2023 ... The selection of solver parameters or initial guesses can be determined by another optimization algorithm to search in among categorical or ...Python equivalence to inline functions or macros. where x is a numpy array of complex numbers. For code readability, I could define a function like. return x.real*x.real+x.imag*x.imag. which is still far faster than abs (x)**2, but it is at the cost of a function call.See doucmentation for the basinhopping algorithm, which also works with multivariate scalar optimization. from scipy.optimize import basinhopping x0 = 0 sol ...Optimization is the act of selecting the best possible option to solve a mathematical problem when choosing from a set of variables. The concept of optimization has existed in mathematics for centuries, but in more recent times, scientists have discovered that other scientific disciplines have common elements, so the idea of optimization has carried …Introduction to Mathematical Optimisation in Python. Beginner’s practical guide to discrete optimisation in Python. Zolzaya Luvsandorj. ·. Follow. …torch.optim. torch.optim is a package implementing various optimization algorithms. Most commonly used methods are already supported, and the interface is general enough, so that more sophisticated ones can also be easily integrated in the future.Optimization with PuLP ... , Optimisation Concepts, and the Introduction to Python before beginning the case-studies. For instructions for the installation of PuLP see Installing PuLP at Home. The full PuLP function documentation is available, and useful functions will be explained in the case studies. The case studies are in …Modern Optimization Methods in Python. Highly-constrained, large-dimensional, and non-linear optimizations are found at the root of most of today's forefront ...Python is one of the most popular programming languages in the world. It is known for its simplicity and readability, making it an excellent choice for beginners who are eager to l... sys.flags.optimize gets set to 1. __debug__ is False. asserts don't get executed. In addition -OO has the following effect: sys.flags.optimize gets set to 2. doc strings are not available. To verify the effect for a different release of CPython, grep the source code for Py_OptimizeFlag. POT: Python Optimal Transport. This open source Python library provide several solvers for optimization problems related to Optimal Transport for signal, image processing and machine learning. Website and documentation: https://PythonOT.github.io/. POT provides the following generic OT solvers (links to examples):Bayesian optimization is a machine learning based optimization algorithm used to find the parameters that globally optimizes a given black box function. There are 2 important components within this algorithm: The black box function to optimize: f ( x ). We want to find the value of x which globally optimizes f ( x ).. Aug 19, 2023 · Python Code OptimizatioMar 14, 2024 · Scikit-Optimize. Scikit-Optimize, or skopt, is a s scipy.optimize.OptimizeResult# class scipy.optimize. OptimizeResult [source] #. Represents the optimization result. Notes. Depending on the specific solver being used, OptimizeResult may not have all attributes listed here, and they may have additional attributes not listed here. Since this class is essentially a subclass of … The scipy.optimize.fmin uses the Nelder-Mead a SciPy is a Python library that is available for free and open source and is used for technical and scientific computing. It is a set of useful functions and mathematical methods created using Python’s NumPy module. ... Import the optimize.linprog module using the following command. Create an array of the …scipy.optimize.brute# scipy.optimize. brute (func, ranges, args=(), Ns=20, full_output=0, finish=<function fmin>, disp=False, workers=1) [source] # Minimize a function over a given range by brute force. Uses the “brute force” method, i.e., computes the function’s value at each point of a multidimensional grid of points, to find the global minimum of the function. Python is a popular programming language known for its simplicity...
Continue Reading