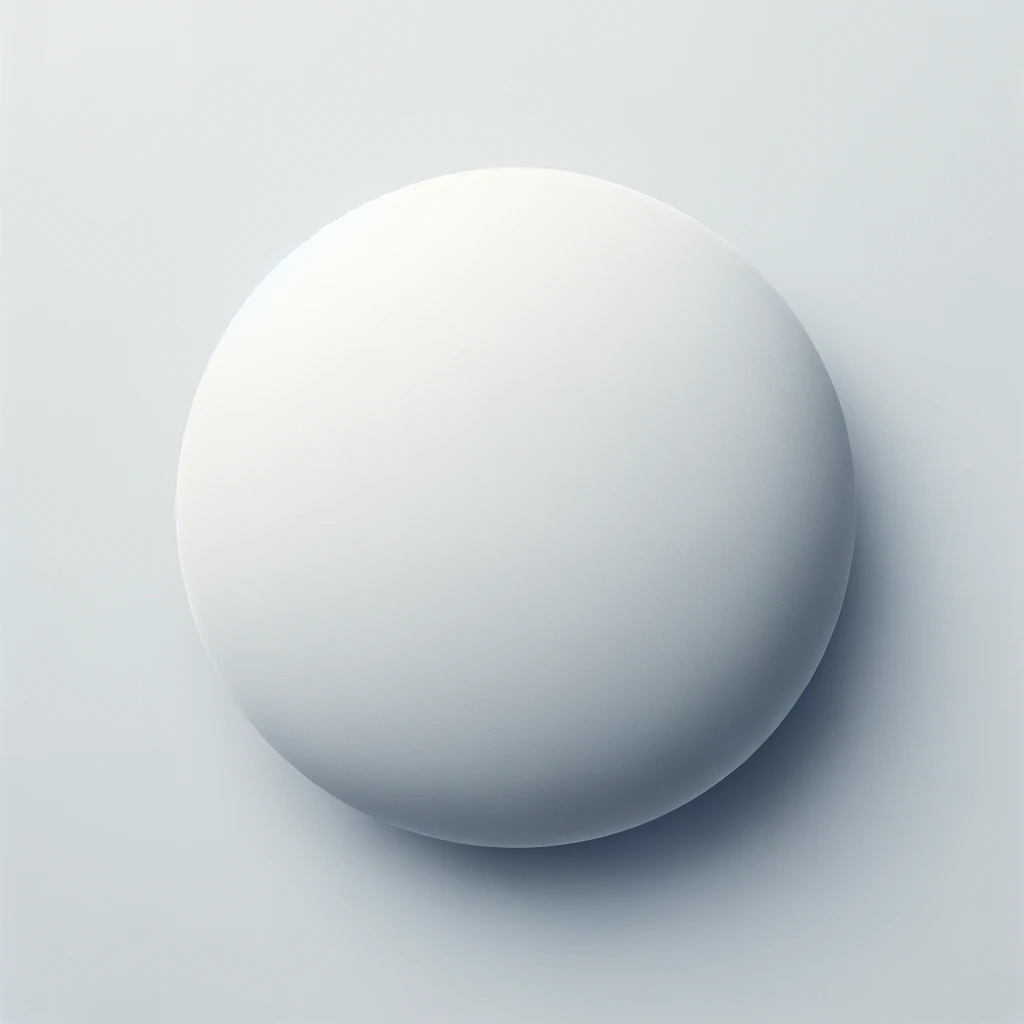
Jan 30, 2024 · Here, I will explain different examples of how to use square brackets to create a list in Python, such as creating a list with an empty list, with different elements of different data types, etc. Below, you can see the code that I have mentioned to create a list in Python using different conditions. According to the Python Documentation: If no argument is given, the constructor creates a new empty list, []. 💡 Tip: This creates a new list object in memory and since we didn't pass any arguments to list (), an empty list will be created. For example: num = list () This empty list will have length 0, as you can see right here:The list is one of the most useful data-type in python. We can add values of all types like integers, string, float in a single list. List initialization can be done using square brackets []. Below is an example of a 1d list and 2d list. As we cannot use 1d list in every use case so python 2d list is used.Values of a: [] Type of a: <class 'list'> Size of a: 0 Python Append to Empty List. In Python, we can create an empty list and then later append items to it, using the Python append() function. Example: In this example, we will first create an empty list in Python using the square brackets and then appended the items to it.Python List comprehension provides a much more short syntax for creating a new list based on the values of an existing list. List Comprehension in Python Example Here is an example of using list comprehension to find the square of the number in Python.Using Python's input() function, you can send user input to a list and save it as a string. After that, the input can be processed to generate a list format.I'm going through a whole bunch of tuples with a many-to-many correlation, and I want to make a dictionary where each b of (a,b) has a list of all the a's that correspond to a b. It seems awkward to test for a list at key b in the dictionary, then look for an a, then append a if it's not already there, every single time through the tuple digesting loop; but I haven't …The list comprehensions actually are implemented more efficiently than explicit looping (see the dis output for example functions) and the map way has to invoke an ophaque callable object on every iteration, which incurs considerable overhead overhead.. Regardless, [[] for _dummy in xrange(n)] is the right way to do it and none of the tiny (if existent at all) speed …In any case, to generate a list of permutations, we can do the following. import trotter. my_permutations = trotter.Permutations(3, [1, 2, 3]) print(my_permutations) for p in my_permutations: print(p) Output: A pseudo-list containing 6 3-permutations of [1, 2, 3]. Lists and tuples are arguably Python’s most versatile, useful data types. You will find them in virtually every nontrivial Python program. Here’s what you’ll learn in this tutorial: You’ll cover the important characteristics of lists and tuples. You’ll learn how to define them and how to manipulate them. Lists and tuples are arguably Python’s most versatile, useful data types. You will find them in virtually every nontrivial Python program. Here’s what you’ll learn in this tutorial: You’ll cover the important characteristics of lists and tuples. You’ll learn how to define them and how to manipulate them. Create a List with list comprehension in Python. List comprehension offers a shorter syntax when we want to create a new list based on the values of an existing list. List comprehension is a sublime way to define and build lists with the help of existing lists. In comparison to normal functions and …Creating dictionary of lists using List Comprehension In list comprehension we iterate over the values '1' , '2' , and '3' , and for each value, it creates a key-value pair in the dictionary. The key is the current value, and the value is a range of integers starting from the value converted to an integer and ending …If you are running Python 3.4+, you can use the venv module baked into Python: python -m venv <directory>. This command creates a venv in the specified directory and copies pip into it as well. If you’re unsure what to call the directory: venv is a commonly seen option; it doesn’t leave anyone guessing what …A more useful way of creating lists within lists is to use a loop. We used the enumerate function on the addresses list so that we would have the current index ...Code Output: This example generates an array of numbers from 0 to 1 (inclusive) with a step size of 0.1. How To Create a List of Numbers from 1 to N in Python Using list() and map(). Another approach to generating lists of numbers in Python involves using the built-in list() function along with the map() function. This combination provides …Example 1: Create lists from string, tuple, and list. # empty list print(list()) # vowel string . vowel_string = 'aeiou' print (list(vowel_string)) # vowel tuple . vowel_tuple = ('a', 'e', 'i', 'o', …Use the append () Function to Create a List of Lists in Python. We can add different lists to a common list using the append () function. It adds the list as an element to the end of the list. The following code will explain this. l1 = [1, 2, 3] l2 = [4, 5, 6] l3 = [7, 8, 9] lst = []Strings are reducible to smaller parts—the component characters. It might make sense to think of changing the characters in a string. But you can’t. In Python, strings are also immutable. The list is the first mutable data type you have encountered. Once a list has been created, elements can be added, deleted, shifted, and …Method 3: Create a matrix in Python using matrix () function. Use the numpy.matrix () function to create a matrix in Python. We can pass a list of lists or a string representation of the matrix to this function. Example: Let’s create a 2*2 matrix in Python. import numpy as np.Ok there is a file which has different words in 'em. I have done s = [word] to put each word of the file in list. But it creates separate lists (print s returns ['it]']['was']['annoying']) as I mentioned above. I want to merge all of them in one list. –Python is a popular programming language known for its simplicity and versatility. Whether you’re a seasoned developer or just starting out, understanding the basics of Python is e...Dec 9, 2018 · Iterate over a list in Python; Python - Find Index containing String in List ... Creating a multidimensional list with all zeros: # Python program to create a m x n ... list() Syntax · list() Parameters · list() Return Value · Example 1: Create lists from string, tuple, and list · Example 2: Create lists from set and di... Python provides a method called .append () that you can use to add items to the end of a given list. This method is widely used either to add a single item to the end of a list or to populate a list using a for loop. Learning how to use .append () will help you process lists in your programs. depending on how you use it, it may be better to omit the list part (or for python 2: use xrange). e.g if you iterate once through every member of the range in a for loop. Share. Improve this answer. ... How do I create a list with the number I want in a loop? 0. how can ı make a list consist of integers from given list. 0.The files required in to-do list project are: tasks.txt – The text file where all our tasks will be stored. main.py – The python script file. Here are the steps you will need to execute to build this python project: Importing all the necessary libraries. Initializing the window and placing all the components in it.Creating a list in python is very simple. You can create an empty list L like this. # This is a python list L that has no items stored. L = [] Lists in python are declared using square brackets. What goes inside these brackets is a comma separated list of items. If no items are provided, an empty list is created.Python’s enumerate () has one additional argument that you can use to control the starting value of the count. By default, the starting value is 0 because Python sequence types are indexed starting with zero. In other words, when you want to retrieve the first element of a list, you use index 0: Python.Jan 19, 2023 ... 1 Answer 1 ... Dictionaries are iterable via keys. ... If the columns in the file are separated by a fixed separator (like a tab or a space), then ...Mar 9, 2024 ... Using For Loop · Initialize an empty list called number_list . · Use the range() function to create a range of numbers from 1 to N+1. · Inside&...Note that this converts the values from whatever numpy type they may have (e.g. np.int32 or np.float32) to the "nearest compatible Python type" (in a list). If you want to preserve the numpy data types, you could call list() on your array instead, and you'll end up with a list of numpy scalars. (Thanks to Mr_and_Mrs_D for pointing that out in a ...Python | Remove consecutive duplicates from list; Python | Make a list of intervals with sequential numbers; Python | Perform append at beginning of list; Python | Intersection of two nested list; Python | Ways to shuffle a list; Python | Select random value from a list; Python | Number of values greater than K in …So you just need a class of object that contains a reference to the original sequence, and a range. Here is the code for such a class (not too big, I hope): class SequenceView: def __init__(self, sequence, range_object=None): if range_object is None: range_object = range(len(sequence)) self.range = range_object. Be aware, that in Python 3.x map() does not return a list (so you would need to do something like this: new_list = list(map(my_func, old_list))). Filling other list using simple for ... in loop. Alternatively you could use simple loop - it is still valid and Pythonic: new_list = [] for item in old_list: new_list.append(item * 10) Generators Python is a popular programming language known for its simplicity and versatility. Whether you’re a seasoned developer or just starting out, understanding the basics of Python is e...Mar 25, 2022 · Create a List of Lists in Python. To create a list of lists in python, you can use the square brackets to store all the inner lists. For instance, if you have 5 lists and you want to create a list of lists from the given lists, you can put them in square brackets as shown in the following python code. Mar 7, 2024 ... In this tutorial, we will explore ways to Create, Access, Slice, Add, Delete Elements to a Python List along with simple examples.Are you looking to enhance your programming skills and boost your career prospects? Look no further. Free online Python certificate courses are the perfect solution for you. Python...Dec 27, 2023 · Python List comprehension provides a much more short syntax for creating a new list based on the values of an existing list. List Comprehension in Python Example. Here is an example of using list comprehension to find the square of the number in Python. The files required in to-do list project are: tasks.txt – The text file where all our tasks will be stored. main.py – The python script file. Here are the steps you will need to execute to build this python project: Importing all the necessary libraries. Initializing the window and placing all the components in it.In Python, “strip” is a method that eliminates specific characters from the beginning and the end of a string. By default, it removes any white space characters, such as spaces, ta...Use the append () Function to Create a List of Lists in Python. We can add different lists to a common list using the append () function. It adds the list as an element to the end of the list. The following code will explain this. l1 = [1, 2, 3] l2 = [4, 5, 6] l3 = [7, 8, 9] lst = []A Python linked list is an abstract data type in Python that allows users to organize information in nodes, which then link to another node in the list. This makes it easier to insert and remove information without changing the index of other items in the list. You want to insert items easily in between other items.Oct 31, 2008 · The append () method adds a single item to the end of the list. The extend () method takes one argument, a list, and appends each of the items of the argument to the original list. (Lists are implemented as classes. “Creating” a list is really instantiating a class. How to Create a List in Python. You can create a list in Python by separating the elements with commas and using square brackets []. Let's create an …Aug 14, 2023 ... You can create an empty list in Python using either square brackets [] or the list() function. An empty list can be used as a placeholder for ...0. Get a list of number as input from the user. This can be done by using list in python. L=list(map(int,input(),split())) Here L indicates list, map is used to map input with the position, int specifies the datatype of the user input which is in integer datatype, and split () is used to split the number based on space.Because you're appending empty_list at each iteration, you're actually creating a structure where all the elements of imp_list are aliases of each other. E.g., if you do imp_list[1].append(4), you will find that imp_list[0] now also has that extra element. So, instead, you should do imp_list.append([]) and make each …Dec 6, 2023 ... To create a list of Tuples in Python, you can use direct initialization by creating a list of tuples and specifying the tuples within square ...@Robino was suggesting to add some tests which make sense, so here is a simple benchmark between 3 possible ways (maybe the most used ones) to convert an iterator to a list: by type constructor. …You can make a shorter list in Python by writing the list elements separated by a comma between square brackets. Running the code squares = [1, 4, 9, 16, ...Python is one of the most popular programming languages in today’s digital age. Known for its simplicity and readability, Python is an excellent language for beginners who are just...Apr 30, 2023 · Creating a list of lists in python is a little tricky. In this article, we will discuss 4 different ways to create and initialize list of lists. Wrong way to create & initialize a list of lists in python. Let’s start from basic, quickest way to create and initialize a normal list with same values in python is, Pandas is great for time series in general, and has direct support for date ranges.. For example pd.date_range():. import pandas as pd from datetime import datetime datelist = pd.date_range(datetime.today(), periods=100).tolist() It also has lots of options to make life easier.Dec 4, 2012 · a = ' ' + string.ascii_uppercase. # if start > end, then start from the back. direction = 1 if start < end else -1. # Get the substring of the alphabet: # The `+ direction` makes sure that the end character is inclusive; we. # always need to go one *further*, so when starting from the back, we. # need to substract one. Pandas is great for time series in general, and has direct support for date ranges.. For example pd.date_range():. import pandas as pd from datetime import datetime datelist = pd.date_range(datetime.today(), periods=100).tolist() It also has lots of options to make life easier.A Python list is a convenient way of storing information altogether rather than individually. As opposed to data types such as int, bool, float, str, a list is a compound data type where you can group values together. In Python, it would be inconvenient to create a new python variable for each data point you collected.Jun 29, 2021 ... In this Python list tutorial, you will learn what is a list in Python and how to use list Python. All the concepts of list are disucseed in ...Python Generator Expression. In Python, a generator expression is a concise way to create a generator object. It is similar to a list comprehension, but instead of creating a list, it creates a generator object that can be iterated over to produce the values in the generator. Generator Expression Syntax. A generator expression has the following ...Code Output: This example generates an array of numbers from 0 to 1 (inclusive) with a step size of 0.1. How To Create a List of Numbers from 1 to N in Python Using list() and map(). Another approach to generating lists of numbers in Python involves using the built-in list() function along with the map() function. This combination provides …You probably want to create a list in your function, use the yield keyword, or use the built in list function.. def generateNumberList(num): myList = [] for i in range(num): myList.append(i) #Notice that your return the list you've created rather #than each individaul integer return myList print generateNumberList(10) def generateNumberList2(num): for i in range(10): …. What I want is to add the incremental value to the step to beSo you just need a class of object that contain What I want is to add the incremental value to the step to become the new step value p.e. step = 1+1 =2, new step =2, new step = 3, new step = 4 etc. Yes increment the step by one on each iteration. Either use a while loop, or a generator (generators can store state of step, whereas iterators can't) as per the top-two answers here. Python List insert() Python List Comprehension. List comprehensio Python offers the following list functions: sort (): Sorts the list in ascending order. type (list): It returns the class type of an object. append (): Adds a single element to a list. extend (): Adds multiple elements to a list. index (): Returns the first appearance of the specified value. What I want is to add the incremental value to the step to become th...
Continue Reading